FrankenNXT – A Frankenstein Story
I rescued an old Lego NXT robot from the dump. Someone thought that an old pile of parts like this had no value.

After cleaning it up and inserting new batteries, not surprisingly, the brick would not power up. The electronics were dead.
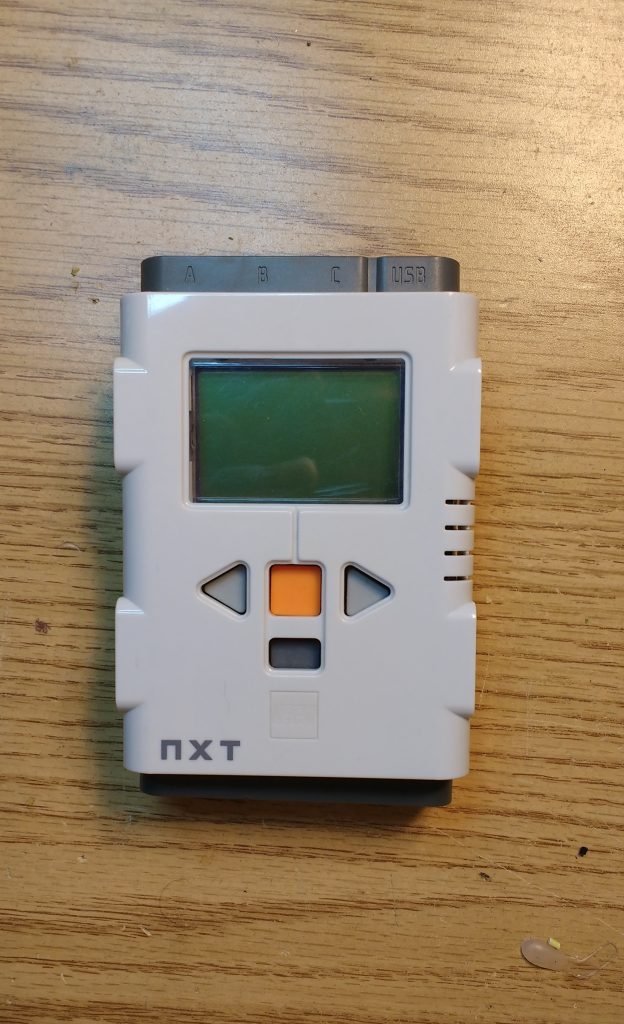
The good news was that the three motors were working fine, so I set about turning this old corpse from the bone yard into a robot again.
I stripped the broken brick for parts.

I soldered wires to the terminal connectors. I then encased each one in a bit of hot glue so the delicate connections would not break.

The motor connectors use all six wires. Motor positive and ground, rotary encoder 5V and ground, and two rotary encoder signal wires.
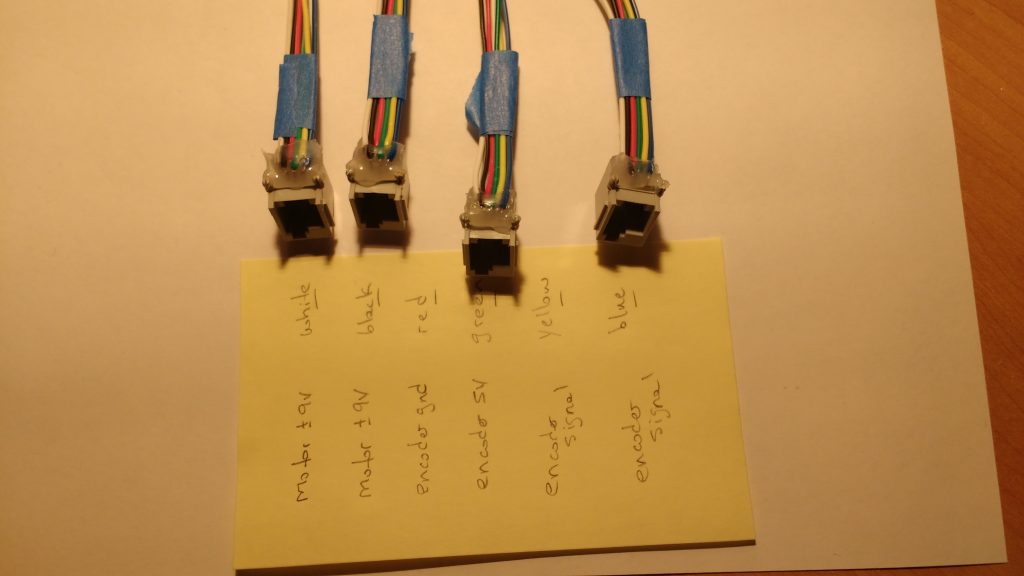
The sensor connectors use only four wires. Two wires are I2C data and clock, and the other two are I2C device 5V and ground.

The connectors fit back into the empty shell of the original brick.

I plugged everything into a bread board to check power consumption and otherwise debug the system.

After confirming that I could turn the motors and nothing was on fire, I worked on getting it assembled and into the case. I had to make room for a buck converter to step down the 9V from 6 AA batteries to the 5V required to run the Raspberry Pi and power the I2C devices in the sensor ports.

A voltage divider is required to get the 5V motor encoder signal down to the 3V3 used by the Raspberry Pi GPIO. This is a simple matter of including two resistors in the circuit for each of the motor encoder signal wires.

I somehow managed to wiggle all the wires into place and everything fit inside the NXT brick shell.

I replaced the brain with a Raspberry pi and an Adafruit motor hat.

The Adafruit motor hat has a bit of space for extra connections, so I added a MCP23008 chip to provide an additional 8 input/output pins. I also included four additional connectors for I2C devices.
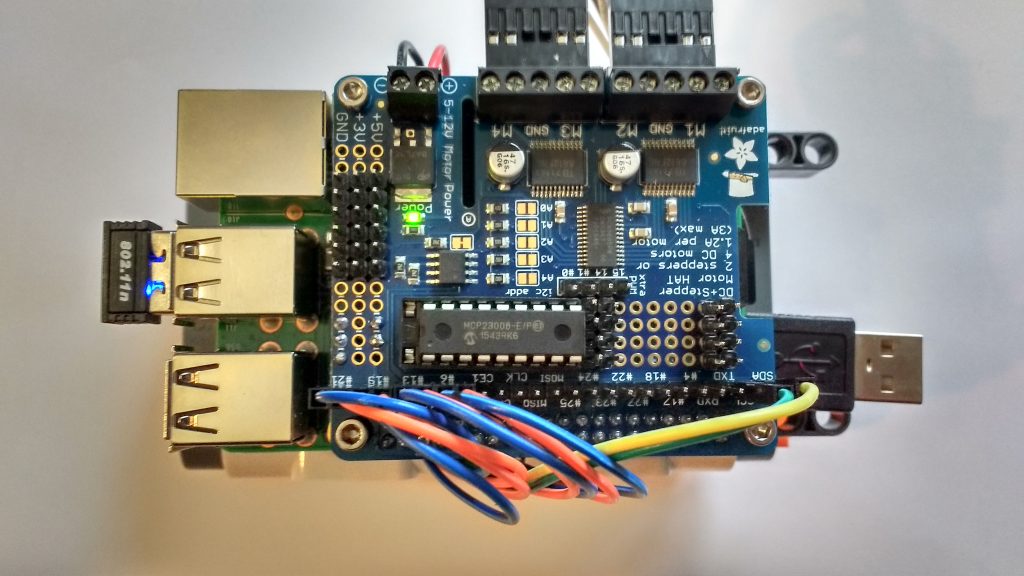
I energized the system with 1.21 gigawatts in the dead of a stormy night.

FrakenNXT was born.
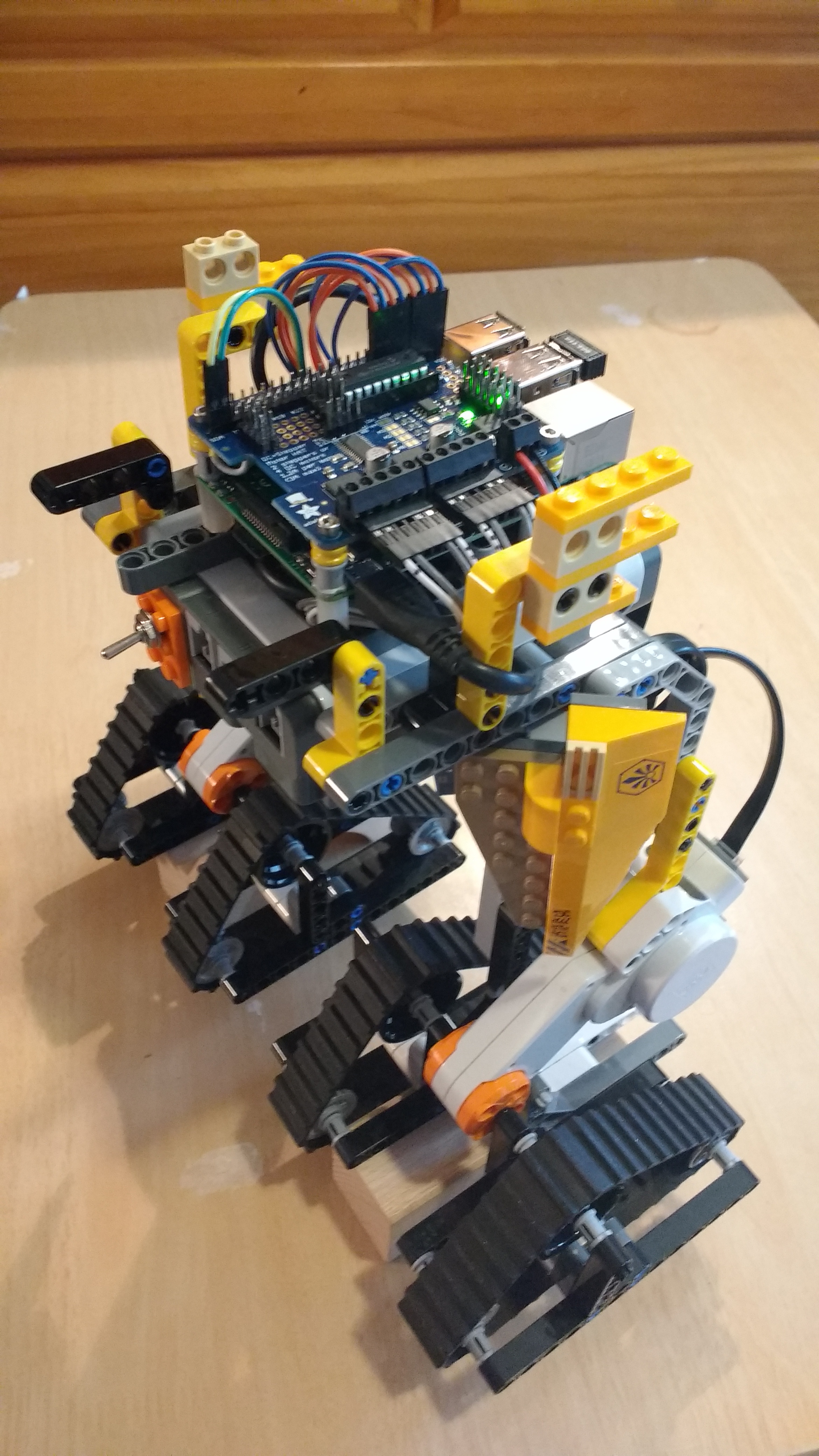
I wanted to show off my creation, so the kids and I went on to build a Lego Chima themed robot on the frame (it so happened that we had a lot of Chima sets around from when the kids were younger).
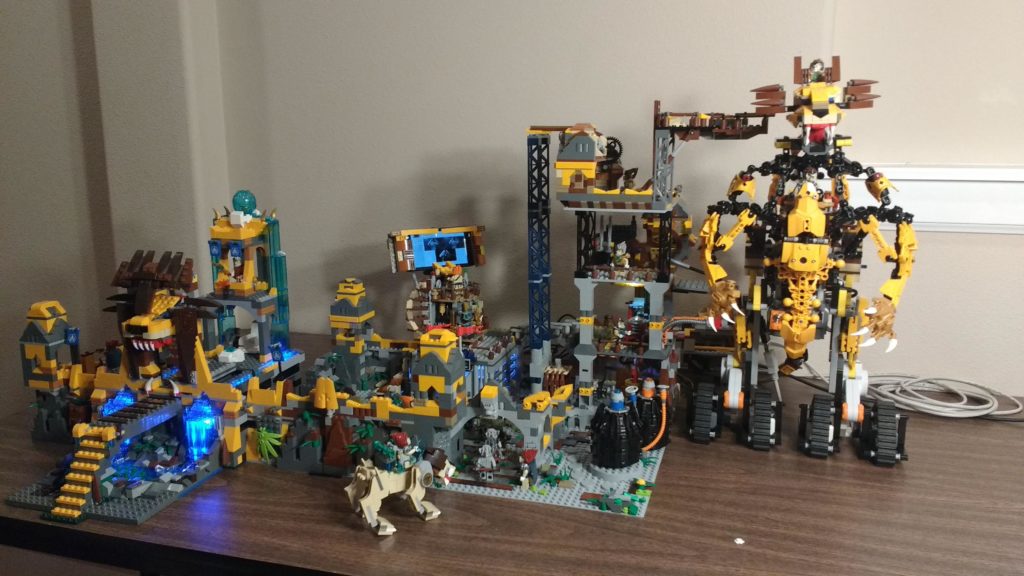
We took it to the Bricks Cascade Lego convention in Portland Oregon, and put it on display with some of our other robotic creations.

A Raspberry Pi camera was added for a simple motion detection demonstration.
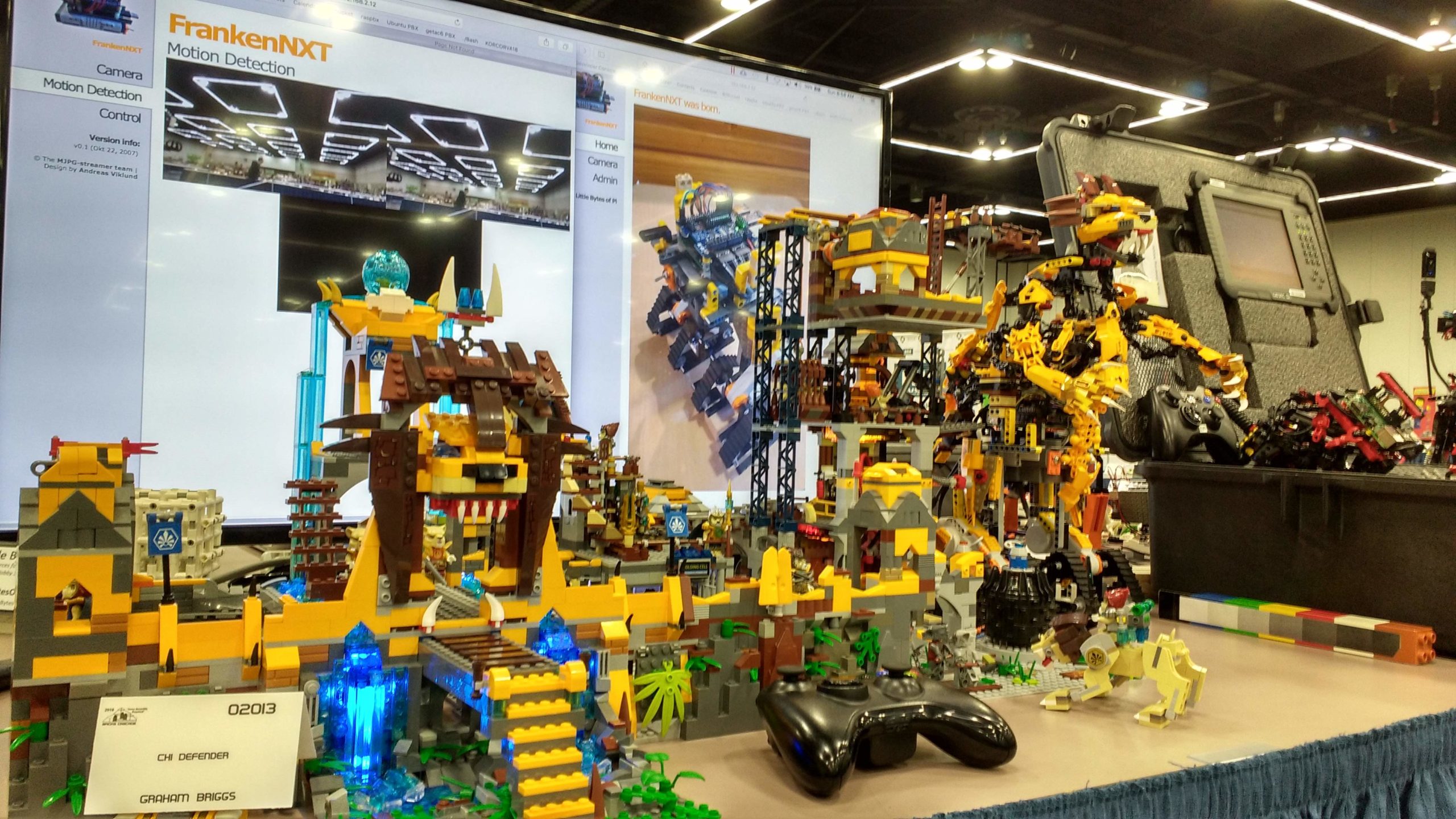
See our FrankenNXT page for details about the software we wrote to control the robot with an Xbox joystick